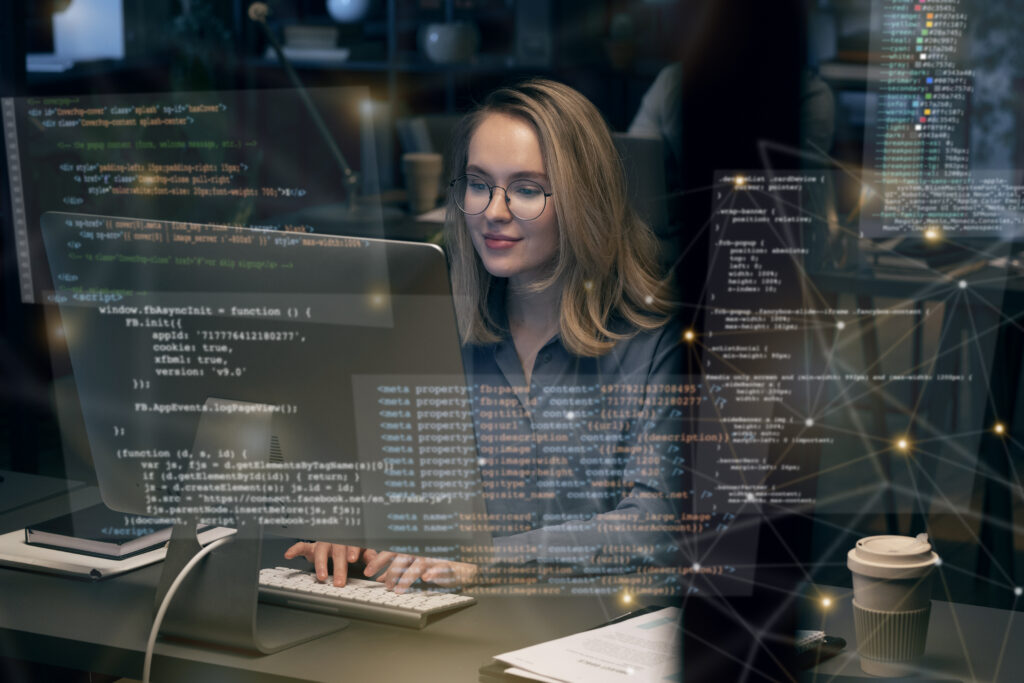
Welcome to our comprehensive tutorial on Numpy Array, a powerful tool for efficient data manipulation in Python. Whether you’re a beginner or an experienced data analyst, this tutorial will guide you through the essential concepts and techniques of Numpy Array, helping you become a master in no time. Let’s dive in!
What is Numpy Array?
Numpy is a Python library that provides support for large, multi-dimensional arrays and matrices. Numpy Arrays are homogenous and offer efficient storage and manipulation of numerical data. To get started, let’s install Numpy using pip:
pip install numpy
Creating Numpy Arrays
You can create Numpy Arrays from Python lists or tuples using the np.array()
function. Let’s create a simple array:
import numpy as np
# Create 0D Array
arr_0D = np.array(28.3)
# Create 1D Array
arr_1D = np.array([28.3, 28.6, 29.1, 28.8])
# Create 2D Array
arr_2D = np.array([[28.3, 28.6, 29.1],
[28.8, 28.9,29.2]])
# Create 3D Array
arr_3D = np.array([[[28.3, 28.6, 29.1],[28.8, 28.9,29.2]],
[[28.1, 28.4, 29.2],[28.6, 28.8, 29.0]]])
Accessing and Manipulating Array Elements
Numpy Arrays support indexing and slicing operations similar to Python lists. Let’s access specific elements and perform array manipulation:
my_array = np.array([1, 2, 3, 4, 5])
# Accessing elements
print(my_array[0]) # Output: 1
print(my_array[1:3]) # Output: [2 3]
# Modifying elements
my_array[2] = 10
print(my_array) # Output: [1 2 10 4 5]
# Reshaping the array
reshaped_array = my_array.reshape((2, 3))
print(reshaped_array)
Performing Mathematical Operations
Numpy Arrays support efficient element-wise operations and mathematical calculations. Let’s perform some basic operations:
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Element-wise operations
addition = array1 + array2
subtraction = array1 - array2
multiplication = array1 * array2
division = array1 / array2
# Aggregation functions
sum_result = np.sum(array1)
mean_result = np.mean(array2)
max_result = np.max(array1)
print(addition) # Output: [5 7 9]
print(subtraction) # Output: [-3 -3 -3]
print(multiplication) # Output: [4 10 18]
print(division) # Output: [0.25 0.4 0.5]
print(sum_result) # Output: 6
print(mean_result) # Output: 5.0
print(max_result) # Output: 3
Advanced Array Operations
Numpy Arrays offer various advanced operations such as sorting, searching, and filtering. Let’s explore some examples:
array = np.array([3, 1, 2, 5, 4])
# Sorting the array
sorted_array = np.sort(array)
print(sorted_array) # Output: [1 2 3 4 5]
# Searching for elements
indices = np.where(array > 3)
print(indices) # Output: (array([3, 4]),)
# Filtering the array
filtered_array = array[array > 2]
print(filtered_array) # Output: [3 5]
Congratulations! You’ve learned the basics of Numpy Array and its powerful features for efficient data manipulation. Now, you can leverage Numpy to perform complex operations, analyze data, and solve real-world problems. Keep exploring and practicing with Numpy to unlock its full potential in your data analysis journey!
Note: Remember to import the numpy
module (import numpy as np
) at the beginning of your code to use Numpy functions and methods.